How to use¶
Platform support¶
This library does not contain any external dependency and can be used on any platform that supports C++11. It is composed of a header and a static .a libary provided for the following platforms:
Linux
Windows
MacOs apple silicon
Esp32s3
Teensy4.X
Note
If you need a precompiled version for another platform, please contact us at CONTACT . We will be happy to provide it in the next release!
The main function (SRX_MODEL_INS_10_DOF::step()
) has to be called at 500Hz by user application.
Stack usage: 2.948 ko
Global variables (tunable parameters): 1.44 ko
Tip
The possibility to build the library on embedded device and on host computer allows for quick development and easy tuning of the parameters using replays of real data!
Convention¶
For the library to work, datas have to be provided in the following convention:
Accelerometer: accelerations in [g]
Gyrometer: rotations speeds in [°/s]
Magnetometer: magntic field intensities in [Gauss]
Barometer: altitude (oriented in upward direction) in [m]
The orientation chosen is Front-Right-Down (FRD), which mean all sensors data have to be given relative to this orientation to provide expected data. Depending on the sensors placements, mapping will have to be done. For example:
SRX-INS00-DEV:
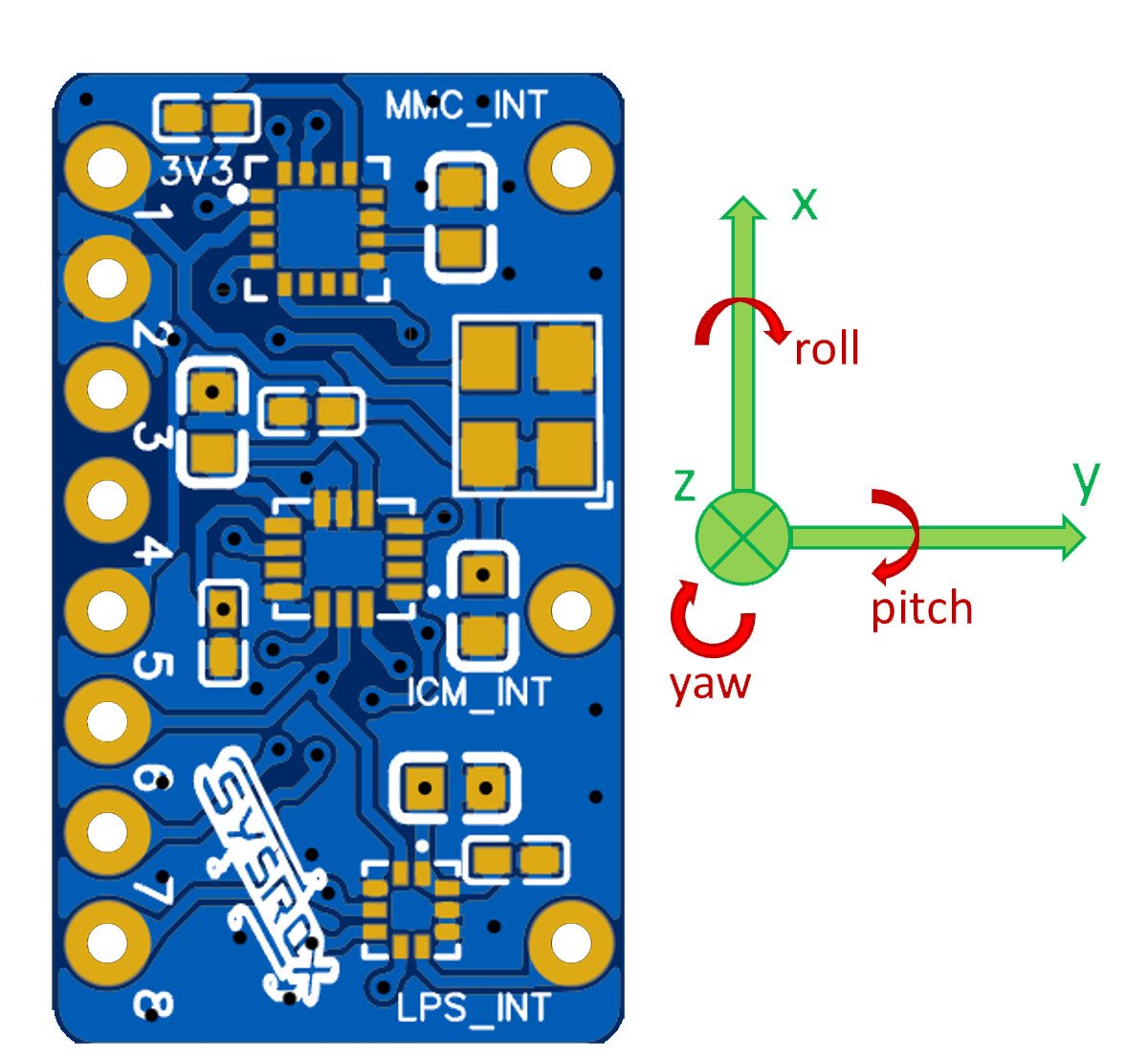
Except for the barometer which is given in upward direction (altitude), all sensors data given following this xyz convention will give orientation following the indicated arrows. If user wants different configuration, he will have to rotate this proposed frame and give sensors data in the new frame.
Usage¶
1. Create object and initialize it¶
Important
API ref: SRX_MODEL_INS_10_DOF
After the inclusion, create the stepable object:
#include "libDM_SRX_10_DOF_interface.hpp"
SRX_MODEL_INS_10_DOF ins;
In any function that is executed one time before loop (setp for arduino …):
ins.initialize();
2. Initialize inputs¶
Important
API ref: SRX_MODEL_INS_10_DOF
, SRX_MODEL_INS_10_DOF::modelInputs
, SRX_MODEL_INS_10_DOF::setInputs()
Before the while loop, create a modelInputs object:
1SRX_MODEL_INS_10_DOF::modelInputs insInputs = SRX_MODEL_INS_10_DOF::modelInputs();
2insInputs.reset = false;
3ins.setInputs(insInputs);
3. Feed the model and step it¶
Important
API ref: SRX_MODEL_INS_10_DOF::setInputs()
, SRX_MODEL_INS_10_DOF::step()
In the while loop, update sensors output, feed the model inputs it and step it at 500Hz:
Note
The mapping proposed is for the SRX-INS00-DEV board.
1// Get sensors data
2icm.readFifo();
3resIMU = icm.getOutput();
4
5mmc.readMag();
6resMAG = mmc.getOutput();
7
8lps.readTemperaturePressureAltitude();
9resBARO = lps.getOutput();
10
11// Feed INS with sensors data
12insInputs.gyroDpsRate[0] = -resIMU.dpsGyroData[1];
13insInputs.gyroDpsRate[1] = -resIMU.dpsGyroData[0];
14insInputs.gyroDpsRate[2] = -resIMU.dpsGyroData[2];
15insInputs.acceleroG[0] = -resIMU.gAccelData[1];
16insInputs.acceleroG[1] = -resIMU.gAccelData[0];
17insInputs.acceleroG[2] = -resIMU.gAccelData[2];
18insInputs.timestampUs = myTimer.returnSystemTimestampUs64();
19
20insInputs.magGauss[0] = -resMAG.GMagData[0];
21insInputs.magGauss[1] = resMAG.GMagData[1];
22insInputs.magGauss[2] = resMAG.GMagData[2];
23insInputs.magTimestampUs = resMAG.usMagTimestamp;
24
25insInputs.barometerAltitudeAmsl = resBARO.mAbsoluteAltitude;
26insInputs.barometerAltitudeRel = resBARO.mRelativeAltitude;
27insInputs.barometerTimestampUs = resBARO.usPressureTimestamp;
28
29// Set inputs and step model
30ins.setInputs(insInputs);
31ins.step(); // Every 2ms
Important
Timestamp is not important for accelerometer and gyrometer as the model runs at the same polling frequency, but is important for magnetometer and barometer to specified their own timestamp as the fusion system will use it to determine if the data has been updated or not.
4. Get outputs¶
After the step, outputs specified in SRX_MODEL_INS_10_DOF::modelOutputs
will be updated and can be retrieved
with copying the pointer content:
1float roll = ins.getOutputs().yawPitchRollLocal[2];
2float pitch = ins.getOutputs().yawPitchRollLocal[1];
3float yawLocalOffsetted = *ins.getOutputs().yawLocalOffsetted;
4std::copy(ins.getOutputs().localToBodyQuat,
5 ins.getOutputs().localToBodyQuat + 4,
6 localQuaternion);
5. Building with your application¶
For this step, the only thing to do is to build .a file with your application. For example, with a simple main.cpp file which include libDM_SRX_10_DOF_interface.hpp:
g++ main.cpp -o libSrx10Dof_windows.a -o myApp.exe
If using platformio, in platformio.ini file add the following lines (for esp32s3):
-L lib/libDM_SRX_INS_10_DOF/src/static_libs
-l Srx10Dof_esp32s3.a
Parameters tuning¶
The topic is detailed in the Tuning section.
Inputs mapping¶
Examples of inputs mapping configurations for SYSROX SRX-INS00-DEV and SRX-INS01-CAS boards:
SRX-INS00-DEV mapping:
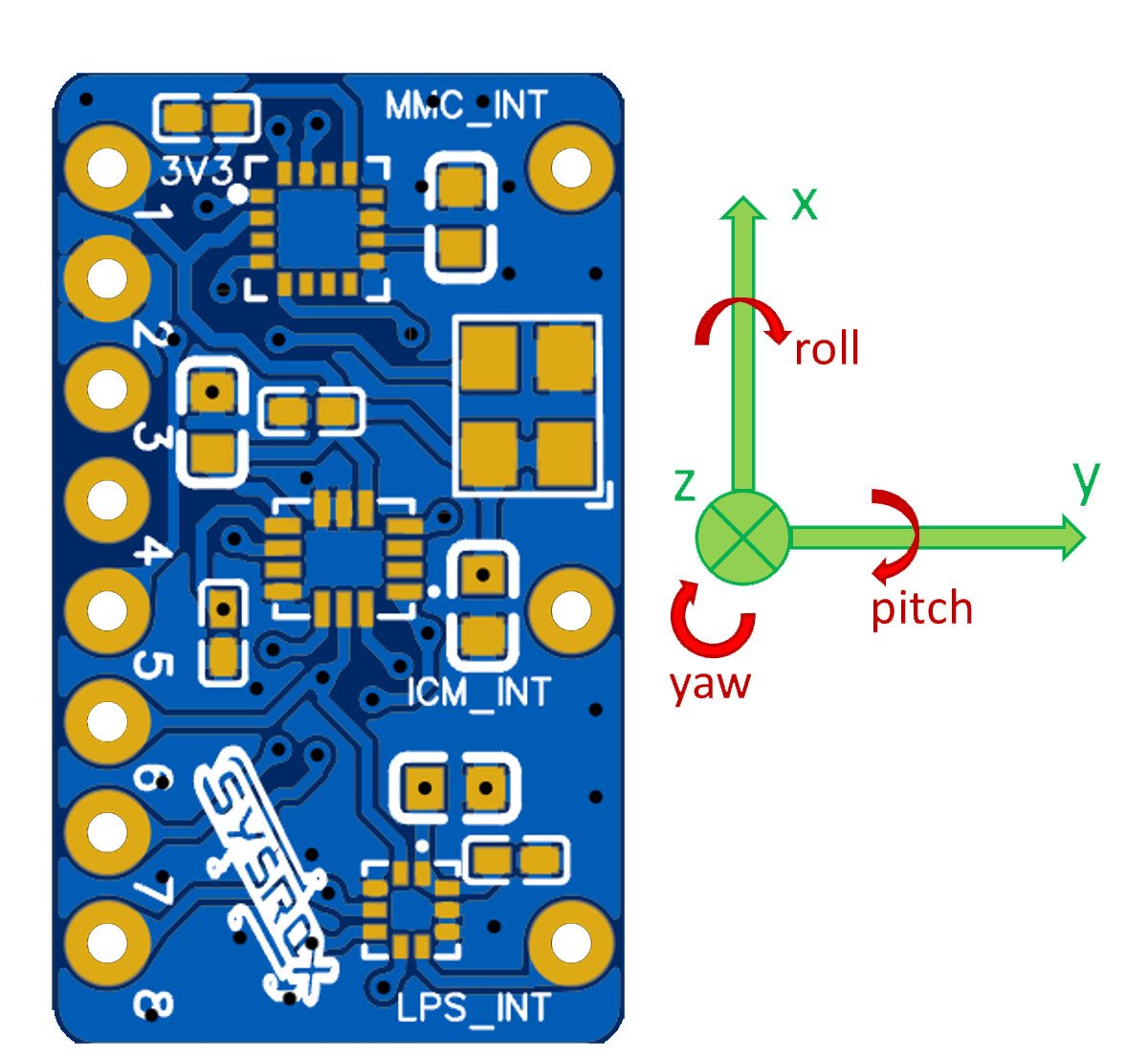
SRX_MODEL_INS_10_DOF::modelInputs insInputs = SRX_MODEL_INS_10_DOF::modelInputs();
GYROMETER:
insInputs.gyroDpsRate[0] = -imuGyroY;
insInputs.gyroDpsRate[1] = -imuGyroX;
insInputs.gyroDpsRate[2] = -imuGyroZ;
ACCELEROMETER:
insInputs.acceleroG[0] = -imuAccY;
insInputs.acceleroG[1] = -imuAccX;
insInputs.acceleroG[2] = -imuAccZ;
MAGNETOMETER:
insInputs.magGauss[0] = -magFX;
insInputs.magGauss[1] = magFY;
insInputs.magGauss[2] = magFZ;
BAROMETER:
insInputs.barometerAltitudeRel = baroRelativeAltitude;
SRX-INS00-DEV mapping:
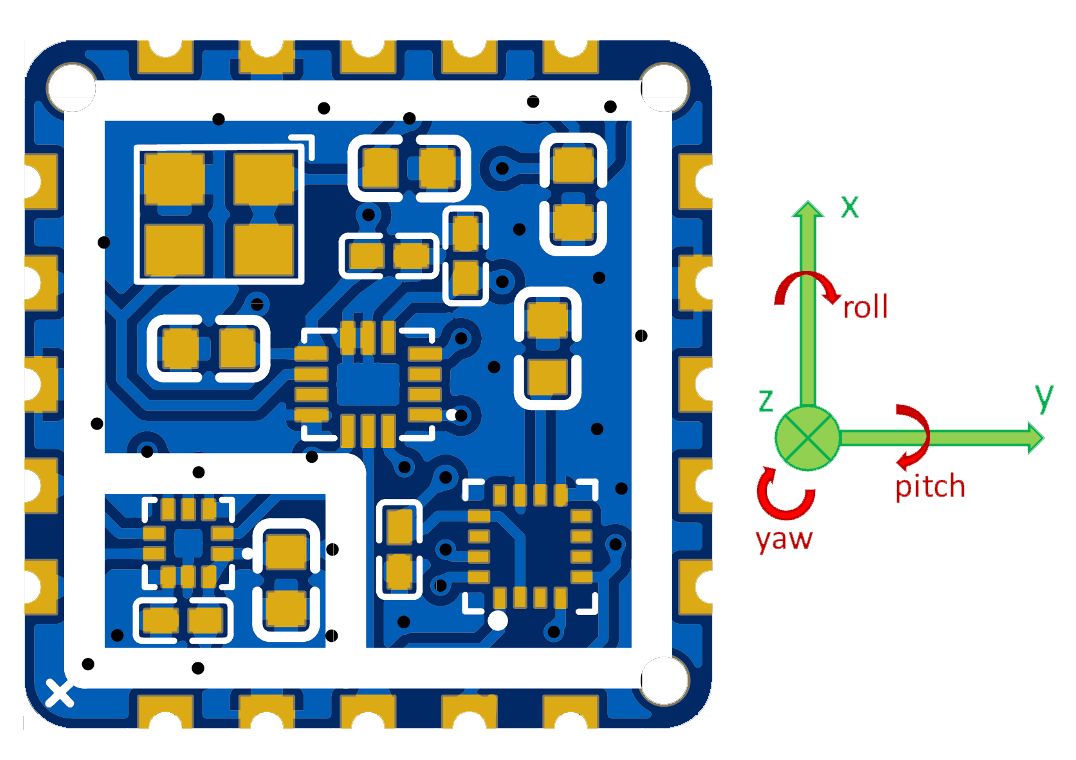
SRX_MODEL_INS_10_DOF::modelInputs insInputs = SRX_MODEL_INS_10_DOF::modelInputs();
GYROMETER:
insInputs.gyroDpsRate[0] = -imuGyroY;
insInputs.gyroDpsRate[1] = -imuGyroX;
insInputs.gyroDpsRate[2] = -imuGyroZ;
ACCELEROMETER:
insInputs.acceleroG[0] = -imuAccY;
insInputs.acceleroG[1] = -imuAccX;
insInputs.acceleroG[2] = -imuAccZ;
MAGNETOMETER:
insInputs.magGauss[0] = magFY;
insInputs.magGauss[1] = magFX;
insInputs.magGauss[2] = magFZ;
BAROMETER:
insInputs.barometerAltitudeRel = baroRelativeAltitude;